Ruby: The Elegant and Dynamic Language
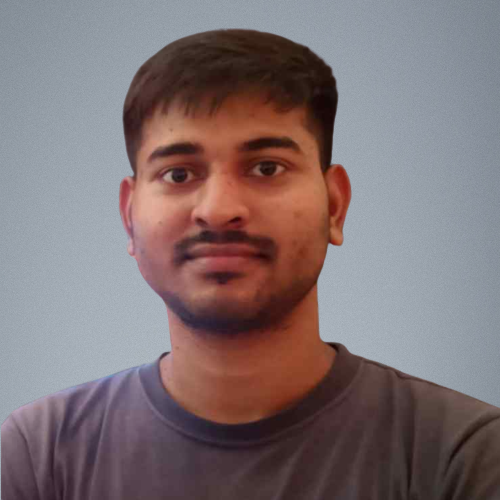
October 24, 2023
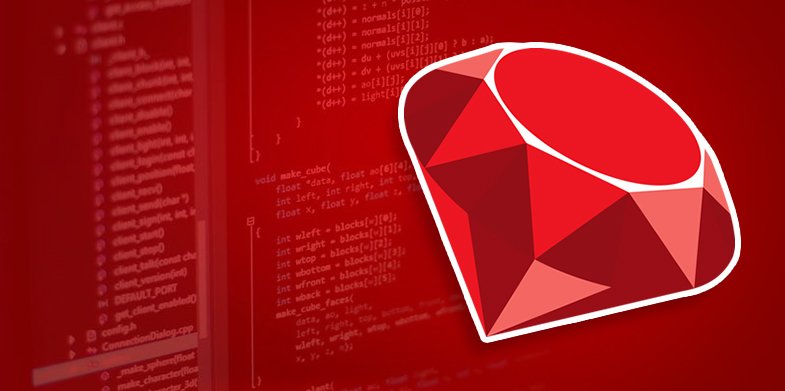
Ruby is a dynamic, object-oriented programming language known for its simplicity, productivity, and elegance. It was created in the mid-1990s by Yukihiro "Matz" Matsumoto, who aimed to design a language that made programmers happy. In this post, we'll explore the many facets of Ruby, from its origins to its use in web development and beyond.
A Language for Humans
Ruby's design philosophy revolves around human-centric coding. Matz's goal was to create a language that was both intuitive and pleasant to write. The result is a language that emphasizes readability and developer happiness.
Here's a simple Ruby program that demonstrates the language's focus on human-friendly code:
class Greeting def initialize(message) @message = message end def greet(name) puts "#{@message}, #{name}!" end end hello = Greeting.new("Hello") hello.greet("Alice")
In this code, we define a Greeting class that initializes with a message and has a greet method. We create an instance of the class and use it to greet a person by name. Ruby's clear and natural syntax makes the code easy to understand.
A Dynamic Language
Ruby is dynamically typed, which means that variable types are determined at runtime. This flexibility makes the language adaptable and suited for rapid development. It also encourages metaprogramming, allowing developers to write code that writes code.
Ruby's dynamic nature can be seen in its support for method definition and modification at runtime. For example, you can add methods to existing classes or change the behavior of methods during execution.
class Dog def bark puts "Woof!" end end fido = Dog.new fido.bark class Dog def bark puts "Ruff!" end end fido.bark
In this code, we initially define a bark method for the Dog class. Later, we redefine the method, changing the dog's bark sound.
The Ruby Community
Ruby's community is known for its friendliness and support for open-source development. The RubyGems package manager and the RubyGems.org repository make it easy to find and share libraries, ensuring that developers have access to a wealth of code to solve various problems.
Ruby's extensive standard library provides built-in modules for tasks like file manipulation, network programming, and regular expressions, making it versatile for a wide range of applications.
Web Development with Ruby on Rails
Ruby on Rails, often referred to simply as Rails, is a popular web application framework built on top of Ruby. Rails follows the convention over configuration (CoC) and don't repeat yourself (DRY) principles, simplifying web application development.
Here's a simple example of a Rails application that displays a "Hello, Ruby on Rails!" message:
app/controllers/welcome_controller.rb
class WelcomeController < ApplicationController def index @message = "Hello, Ruby on Rails!" end end
app/views/welcome/index.html.erb
<h1><%= @message %></h1>
This code demonstrates a basic controller and view in a Ruby on Rails application. The controller handles the logic, and the view displays the message. Rails takes care of routing, database access, and many other aspects, allowing developers to focus on building the application's features.
Conclusion
Ruby is more than just a programming language; it's a philosophy that values human-centric code and developer happiness. Its dynamic nature, metaprogramming capabilities, and vibrant community have made it a compelling choice for various types of projects, from web development to scripting and automation.
Whether you're building web applications with Ruby on Rails, exploring the world of metaprogramming, or simply enjoying the elegance of the language, Ruby provides a joyful and productive experience.
Ruby continues to evolve, with frequent updates and improvements. It remains a valuable tool in the arsenal of developers who appreciate clean, expressive code. So, if you're seeking a language that places the developer's experience at the forefront, Ruby is a language you'll find both elegant and inspiring. Happy coding!
You need to Login to comment.