Rust: Empowering Safe and Efficient Systems Programming
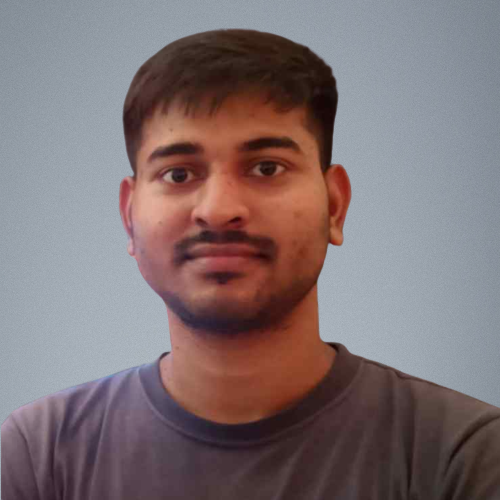
October 26, 2023

Rust is a modern programming language that has taken the world of systems programming by storm. Known for its focus on safety, performance, and a strong community, Rust has become a popular choice for building system-level software. In this post, we'll delve into the many aspects of Rust, from its origins to its unique features.
A Language with a Purpose
Rust was created by Mozilla in 2010 as a response to the shortcomings of C and C++. While these languages have a strong history in systems programming, they suffer from issues like memory safety vulnerabilities, data races, and unpredictable performance.
Rust was designed with the aim of providing the low-level control of C and C++ without sacrificing safety. It achieves this through features like:
-
Ownership and Borrowing: Rust introduces a novel ownership system where each value has a single "owner." Ownership can be transferred or borrowed, ensuring that memory is managed without the risk of leaks or data races.
-
Lifetimes: Lifetimes in Rust allow the compiler to ensure that references remain valid throughout their usage, preventing common programming errors.
-
Zero-cost Abstractions: Rust allows high-level abstractions without sacrificing performance. The ownership system and the borrow checker ensure that abstractions compile down to efficient machine code.
Here's an example of a simple Rust program that demonstrates ownership and borrowing:
fn main() { let mut s = String::from("Hello, "); s.push_str("world!"); println!("{}", s); }
In this code, we create a String variable s, which owns the string data. We then append a string literal to it, demonstrating the concept of ownership and mutability in Rust.
Strong Ecosystem and Community
One of Rust's most impressive aspects is its vibrant and welcoming community. The official package manager, Cargo, has become a shining example of how package management should work. It streamlines project setup, dependencies, and building, making Rust an attractive choice for developers.
The Rust community also embraces open source development, and it hosts a wealth of libraries and frameworks on crates.io. Whether you're building a web application, a game, or an embedded system, you'll find libraries and tools to support your needs.
Here's how you can use the reqwest crate to make an HTTP GET request in Rust:
extern crate reqwest; fn main() -> Result<(), reqwest::Error> { let response = reqwest::blocking::get("https://example.com")?; let body = response.text()?; println!("{}", body); Ok(()) }
In this example, we use the reqwest crate to make an HTTP GET request and print the response body. This demonstrates the ease of using external libraries in Rust projects.
WebAssembly and Beyond
Rust has made significant inroads into web development through WebAssembly (Wasm). With its ability to compile to WebAssembly, Rust enables high-performance applications in the browser, transcending the limitations of traditional JavaScript.
This opens up possibilities for game development, multimedia applications, and even running complex desktop applications in the browser. Rust's growing role in the WebAssembly ecosystem continues to expand its reach.
Here's a simple example of running Rust in the browser using WebAssembly:
// Rust code #[no_mangle] pub fn add(a: i32, b: i32) -> i32 { a + b }
<!-- HTML to load and run the WebAssembly module --> <!DOCTYPE html> <html> <head> <script> async function loadAndRunWasm() { const response = await fetch('your-rust-module.wasm'); const bytes = new Uint8Array(await response.arrayBuffer()); const { instance } = await WebAssembly.instantiate(bytes, {}); const result = instance.exports.add(3, 4); console.log(`3 + 4 = ${result}`); } loadAndRunWasm(); </script> </head> <body> <h1>WebAssembly in Rust</h1> </body> </html>
In this code, we define a simple Rust function that adds two numbers and then load and run it in the browser using WebAssembly.
Safe Concurrency
One of Rust's standout features is its approach to concurrency. The language leverages a "fearless" concurrency model, where threads and data races are caught at compile time. This means you can write concurrent code without the fear of deadlocks and data races.
The async/await syntax, inspired by other modern languages, simplifies asynchronous programming. It allows developers to write asynchronous code that is as easy to understand as synchronous code while benefiting from the performance advantages of non-blocking operations.
Here's an example of using asynchronous code with async/await in Rust:
use tokio::time::Duration; use tokio::task; #[tokio::main] async fn main() { let task1 = task::spawn(async { // Perform asynchronous operations tokio::time::sleep(Duration::from_secs(2)).await; println!("Task 1 is complete."); }); let task2 = task::spawn(async { // Perform other asynchronous operations tokio::time::sleep(Duration::from_secs(1)).await; println!("Task 2 is complete."); }); tokio::join!(task1, task2); }
In this code, we use the tokio library to perform asynchronous operations with async/await. The Rustacean Future
Rust is not just a language; it's a movement that advocates for safer, more efficient systems programming. Its growing popularity has led to its adoption in major projects like the Linux kernel, where it is used for security and performance-critical code.
Rust continues to evolve, with frequent releases adding new features, improvements, and libraries. As the language matures, it's finding its place in more domains, from embedded systems to cloud services.
Conclusion
Rust is more than a programming language; it's a philosophy that places safety and performance at the forefront of systems programming. With its unique ownership system, thriving community, and a bright future, Rust has become the go-to language for developers who demand both power and safety.
If you're looking to explore systems programming or are intrigued by the idea of writing fast, reliable code, Rust is a language you should consider learning. It's not just a language; it's a new way of thinking about systems programming, and it's here to stay.
So, whether you're building the next operating system or a small embedded IoT device, Rust is your ally in the quest for safe and efficient systems programming. Embrace the power of Rust and join the growing community of Rustaceans.
You need to Login to comment.